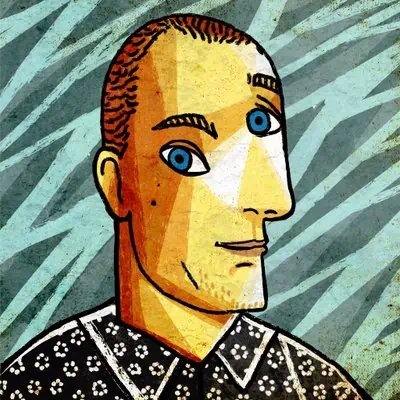
Hi, I'm Carl.
I make distributed systems and developer tools.
A forty-something software engineer, living in Uppsala, Sweden. Aside from the obvious interest in software, I like music and playing the guitar. AGI and climate change are also two things that fascinate me a lot.
I'm currently working as a software engineer at Epic Games. Or, more specifically on the Unreal Engine where I improve developer tooling and surrounding infrastructure for builds.
For a brief overview what I’m currently up to, see my now page.
Occasionally I write posts of what's on my mind, mostly if not only tech related.
I am cgbystrom on most sites and services, and you can find me on Twitter (yeah, yeah.. X), GitHub or LinkedIn and via mail, my alias above at gmail com.